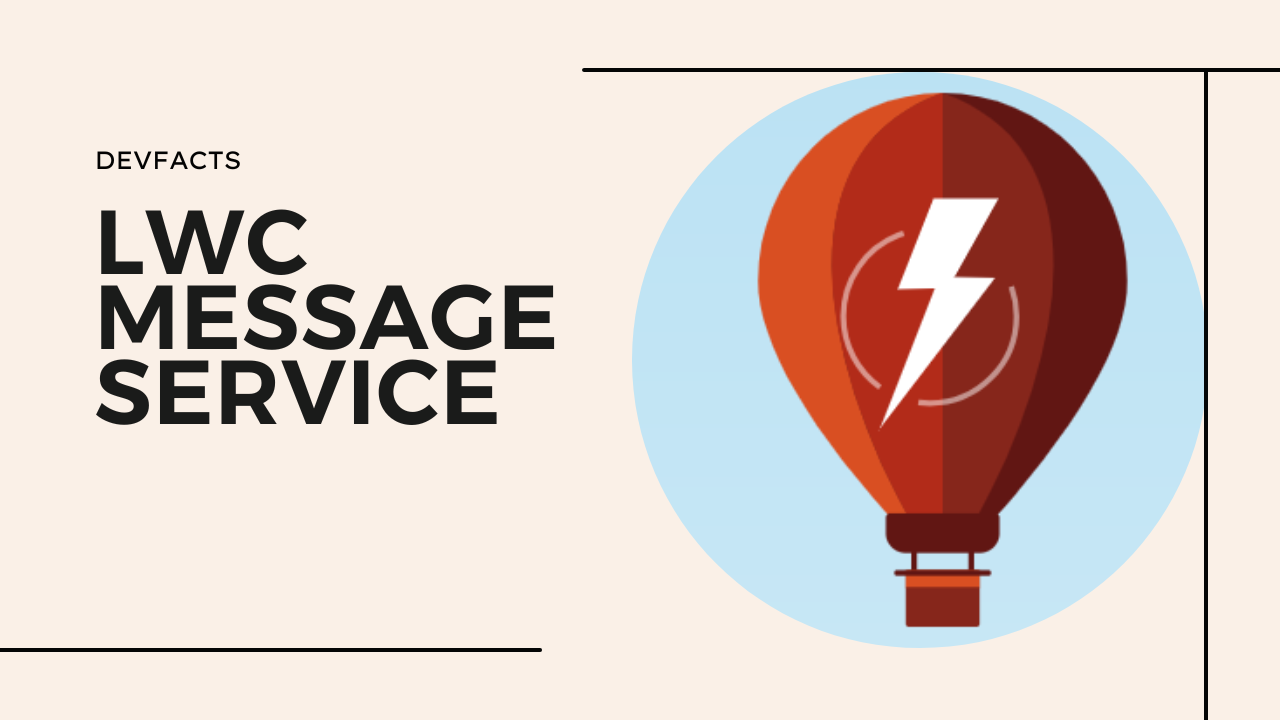
How Lightning Message Service in LWC with Example
The Lightning Message Service (LMS) is a feature of Lightning Web Components (LWC) that allows you to communicate between different components in your Salesforce application. LMS allows you to pass data between components without using global variables or events. Here’s an example of how to use LMS in an LWC component:
import { LightningElement, wire } from 'lwc'; import { CurrentPageReference } from 'lightning/navigation'; import { fireEvent } from 'c/pubsub'; export default class MyComponent extends LightningElement { @wire(CurrentPageReference) pageRef; handleClick() { fireEvent(this.pageRef, 'myevent', { message: 'Hello World!' }); } }
In the above example, we’re using the fireEvent
method from the c/pubsub
module to fire an event called myevent
when the button is clicked. The event is fired with a payload of { message: 'Hello World!' }
. The CurrentPageReference
is used to obtain the current page reference, so that the event can be fired to the current page.
In another component, you can handle the event and receive the message like this:
import { LightningElement, wire } from 'lwc'; import { track } from 'lwc'; import { registerListener, unregisterAllListeners } from 'c/pubsub'; export default class MyComponent2 extends LightningElement { @track message; connectedCallback() { registerListener('myevent', this.handleEvent, this); } disconnectedCallback() { unregisterAllListeners(this); } handleEvent(event) { this.message = event.message; } }
In this example, we’re using the registerListener
method from the c/pubsub
module to register a listener for the myevent
event. The handleEvent
method is called when the event is fired, and it updates the message
property with the value passed in the event payload.
The disconnectedCallback
is used to unregister the listener when the component is destroyed.
This is just a basic example of how to use the Lightning Message Service (LMS) in LWC, You can use it to pass data between components, to notify other components of an event or to share data between different parts of the application.